# baseAt
# Description
lodash at
的基本实现, baseAt
可以根据指定的一组属性路径 paths
(Array
),从 object
中取出属性路径对应的一组值。
# Params
(object, paths)
{Object} object: 源对象
{string[]} paths: 路径数组
# Return
Array
返回取到的值的数组,下标和
paths
一一对应
# Depend
import get from '../get.js'
# Code
function baseAt(object, paths) {
let index = -1
const length = paths.length
const result = new Array(length)
const skip = object == null
while (++index < length) {
result[index] = skip ? undefined : get(object, paths[index])
}
return result
}
# Analyze
- 定义
index
为-1
, 获取到paths
的length
, 定义result
数组, 判断object
是否为null
- 使用
while
循环遍历获取object
的值,并push
到result
中 - 如果
object
为null
,则值为undefined
, 否则使用get
方法,获取对应下标的path
对应的值
# Remark
with i++, before you can increment i under the hood a new copy of i must be created. Using ++i you don't need that extra copy. i++ will return the current value before incrementing i. ++i returns the incremented version i.
使用i++,在对i进行增量操作之前,必须先创建i的新副本。 使用++i,你不需要那份额外的副本。 i++将返回i加1之前的当前值。
关于第二点,
++i
和i++
的性能问题,在搜索了好久之后,并没有得到一个统一的答复,性能问题的回答都是说要区分语言,在js
中,最多的回答是说来自于一篇文章 http://jsperf.com/i-vs-i/2 (opens new window) ,第二点也就是这篇文章的引用, 但是打开时链接失效了,502
, 对比了看了一下ecmascript
的标准实现,发现i++
和++i
在实现上并没有什么不同。所以关于性能问题,可以在查找一些其他资料进行学习
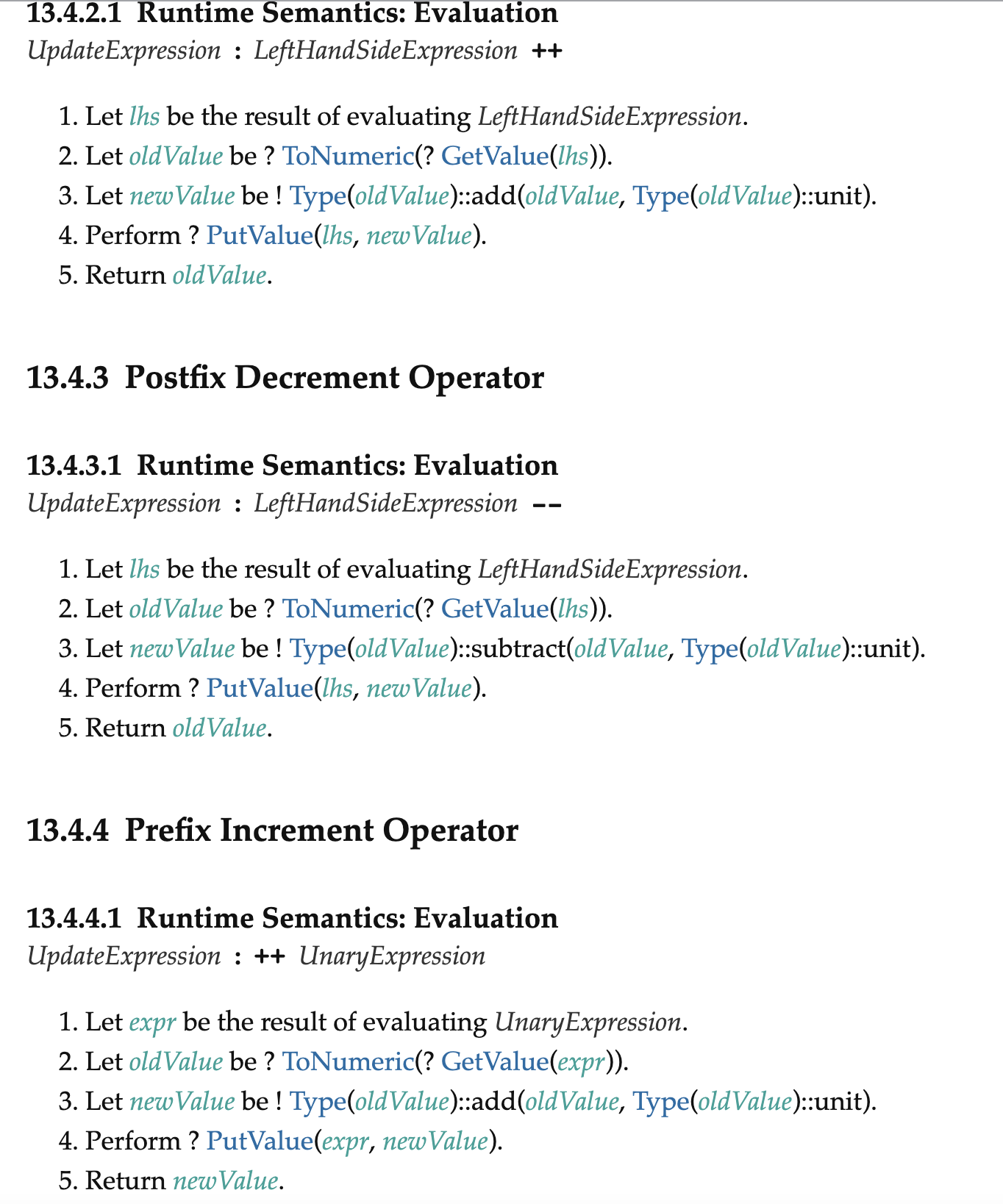
- 参考回答 Is there a performance difference between i++ and ++i in JavaScript? (opens new window)
- 参考回答 Is there a performance difference between i++ and ++i in C? (opens new window)
# Example
var a = {
b: {
c: {
d: 1
},
e: 2
},
g: {
h: 3
}
}
console.log(baseAt(a, ['b.c.d', 'b[e]', 'g["h"]'])) // [1, 2, 3]